How Builders Can Strengthen Their Debugging Capabilities By Gustavo Woltmann
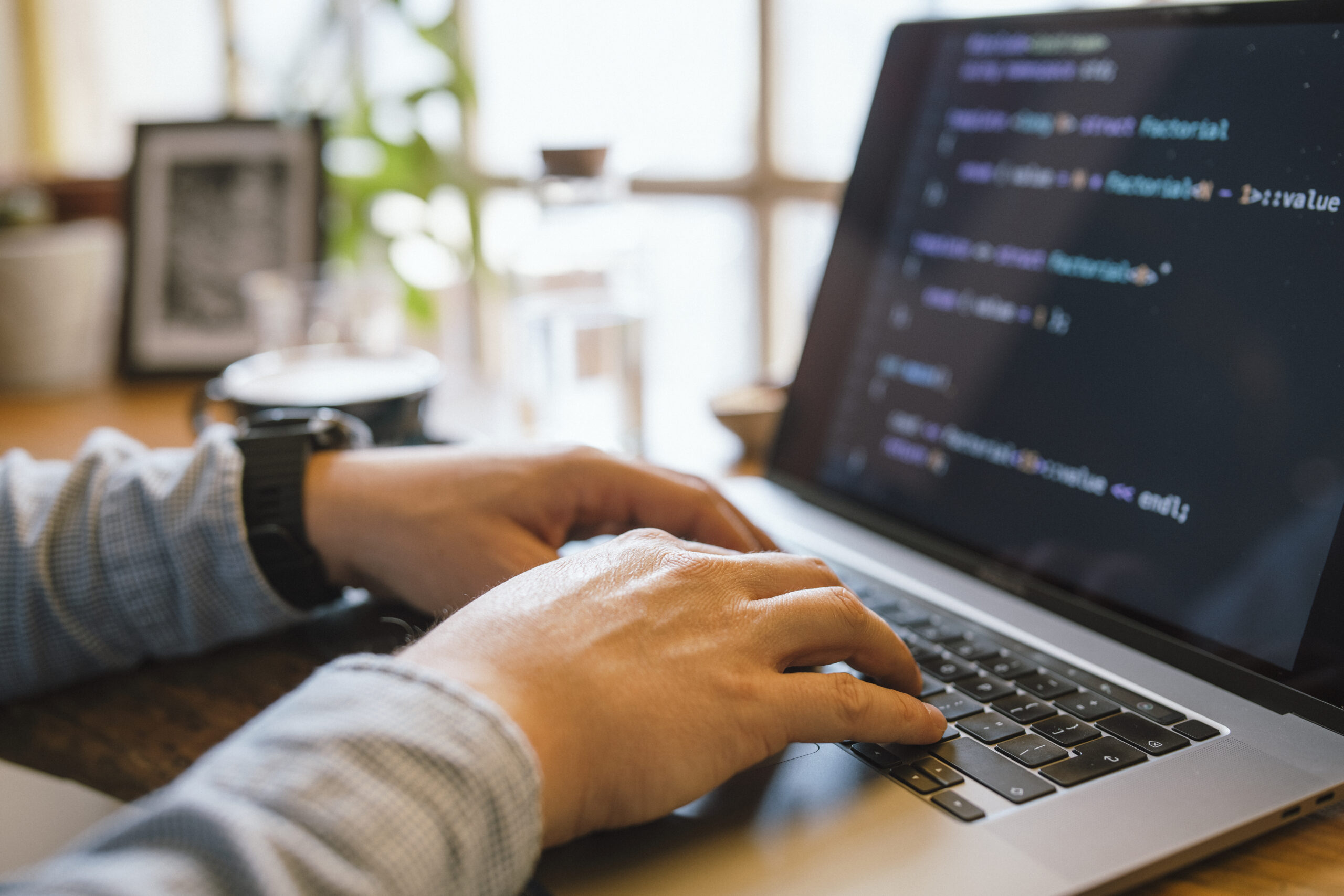
Debugging is Probably the most vital — nonetheless frequently disregarded — techniques inside of a developer’s toolkit. It isn't really just about correcting damaged code; it’s about being familiar with how and why things go Incorrect, and Understanding to Feel methodically to unravel complications efficiently. Whether or not you're a beginner or a seasoned developer, sharpening your debugging capabilities can preserve hrs of annoyance and considerably transform your productiveness. Here i will discuss quite a few procedures that can help builders degree up their debugging sport by me, Gustavo Woltmann.
Grasp Your Resources
Among the fastest means builders can elevate their debugging expertise is by mastering the equipment they use daily. Whilst writing code is a person Component of growth, realizing the way to interact with it effectively in the course of execution is Similarly crucial. Contemporary progress environments arrive equipped with highly effective debugging capabilities — but many builders only scratch the area of what these equipment can do.
Acquire, by way of example, an Built-in Growth Natural environment (IDE) like Visible Studio Code, IntelliJ, or Eclipse. These resources allow you to established breakpoints, inspect the value of variables at runtime, step as a result of code line by line, and in many cases modify code about the fly. When utilized the right way, they Enable you to notice particularly how your code behaves all through execution, which can be a must have for tracking down elusive bugs.
Browser developer resources, like Chrome DevTools, are indispensable for entrance-conclusion developers. They help you inspect the DOM, keep track of community requests, view true-time general performance metrics, and debug JavaScript while in the browser. Mastering the console, resources, and network tabs can switch frustrating UI concerns into manageable duties.
For backend or system-degree builders, applications like GDB (GNU Debugger), Valgrind, or LLDB supply deep Regulate above jogging procedures and memory management. Understanding these instruments can have a steeper Mastering curve but pays off when debugging performance concerns, memory leaks, or segmentation faults.
Outside of your IDE or debugger, come to be comfortable with Edition Regulate techniques like Git to be familiar with code historical past, find the exact second bugs have been launched, and isolate problematic modifications.
In the long run, mastering your applications means going past default options and shortcuts — it’s about creating an intimate understanding of your advancement natural environment to make sure that when challenges arise, you’re not missing in the dead of night. The greater you already know your instruments, the greater time you may shell out resolving the particular challenge in lieu of fumbling by the procedure.
Reproduce the situation
Among the most critical — and often overlooked — steps in helpful debugging is reproducing the condition. In advance of leaping into your code or building guesses, developers require to create a dependable natural environment or circumstance wherever the bug reliably appears. With out reproducibility, correcting a bug will become a match of opportunity, often leading to squandered time and fragile code alterations.
The first step in reproducing a problem is accumulating as much context as possible. Inquire questions like: What steps resulted in the issue? Which environment was it in — development, staging, or output? Are there any logs, screenshots, or error messages? The greater depth you might have, the less difficult it gets to be to isolate the precise disorders under which the bug happens.
When you’ve collected more than enough facts, attempt to recreate the challenge in your local setting. This could indicate inputting exactly the same facts, simulating comparable consumer interactions, or mimicking procedure states. If The problem seems intermittently, think about crafting automated assessments that replicate the edge scenarios or state transitions included. These checks not just enable expose the issue but also avoid regressions Down the road.
Occasionally, The problem may very well be atmosphere-distinct — it'd transpire only on certain working devices, browsers, or under distinct configurations. Using instruments like virtual equipment, containerization (e.g., Docker), or cross-browser screening platforms can be instrumental in replicating this kind of bugs.
Reproducing the challenge isn’t simply a step — it’s a attitude. It requires persistence, observation, plus a methodical tactic. But once you can regularly recreate the bug, you are presently midway to correcting it. Which has a reproducible scenario, You should use your debugging resources a lot more properly, take a look at potential fixes safely, and communicate more clearly together with your team or customers. It turns an summary grievance into a concrete challenge — and that’s where developers prosper.
Examine and Understand the Error Messages
Error messages are often the most valuable clues a developer has when something goes wrong. Rather than looking at them as irritating interruptions, developers should learn to take care of mistake messages as direct communications from your method. They usually tell you exactly what transpired, the place it occurred, and occasionally even why it transpired — if you know the way to interpret them.
Get started by looking at the concept cautiously As well as in entire. Several builders, particularly when beneath time stress, look at the primary line and instantly get started generating assumptions. But deeper during the mistake stack or logs could lie the true root bring about. Don’t just copy and paste mistake messages into engines like google — study and have an understanding of them to start with.
Split the mistake down into elements. Can it be a syntax error, a runtime exception, or simply a logic error? Will it position to a specific file and line range? What module or perform activated it? These concerns can tutorial your investigation and stage you towards the dependable code.
It’s also helpful to grasp the terminology of the programming language or framework you’re employing. Mistake messages in languages like Python, JavaScript, or Java usually observe predictable patterns, and Finding out to recognize these can considerably speed up your debugging approach.
Some faults are vague or generic, and in All those instances, it’s very important to examine the context through which the mistake happened. Verify relevant log entries, enter values, and up to date improvements in the codebase.
Don’t neglect compiler or linter warnings both. These generally precede larger concerns and supply hints about probable bugs.
Finally, error messages are certainly not your enemies—they’re your guides. Learning to interpret them properly turns chaos into clarity, aiding you pinpoint troubles more quickly, lessen debugging time, and turn into a more effective and assured developer.
Use Logging Properly
Logging is The most highly effective instruments inside of a developer’s debugging toolkit. When made use of effectively, it provides actual-time insights into how an application Gustavo Woltmann AI behaves, aiding you realize what’s taking place beneath the hood with no need to pause execution or stage throughout the code line by line.
A very good logging system starts off with recognizing what to log and at what amount. Prevalent logging degrees incorporate DEBUG, Data, WARN, Mistake, and FATAL. Use DEBUG for comprehensive diagnostic info throughout development, INFO for typical situations (like prosperous start-ups), Alert for likely concerns that don’t break the applying, Mistake for true issues, and FATAL when the system can’t continue on.
Prevent flooding your logs with extreme or irrelevant information. Far too much logging can obscure significant messages and decelerate your method. Target important situations, condition modifications, enter/output values, and significant selection details as part of your code.
Structure your log messages Obviously and continuously. Incorporate context, like timestamps, ask for IDs, and function names, so it’s much easier to trace concerns in dispersed systems or multi-threaded environments. Structured logging (e.g., JSON logs) might make it even easier to parse and filter logs programmatically.
Through debugging, logs Allow you to keep track of how variables evolve, what situations are achieved, and what branches of logic are executed—all with no halting This system. They’re Specifically useful in output environments in which stepping as a result of code isn’t achievable.
On top of that, use logging frameworks and resources (like Log4j, Winston, or Python’s logging module) that aid log rotation, filtering, and integration with monitoring dashboards.
Finally, smart logging is about equilibrium and clarity. Using a perfectly-believed-out logging tactic, you are able to decrease the time it's going to take to spot difficulties, achieve further visibility into your programs, and Enhance the In general maintainability and reliability of the code.
Assume Similar to a Detective
Debugging is not just a specialized task—it's a kind of investigation. To proficiently identify and repair bugs, developers have to solution the process just like a detective fixing a secret. This mentality assists break down sophisticated troubles into workable pieces and follow clues logically to uncover the basis bring about.
Get started by accumulating proof. Think about the symptoms of the problem: error messages, incorrect output, or overall performance concerns. Similar to a detective surveys against the law scene, obtain just as much suitable facts as you could without the need of leaping to conclusions. Use logs, exam conditions, and person reports to piece together a transparent photograph of what’s occurring.
Following, kind hypotheses. Question oneself: What could possibly be leading to this habits? Have any alterations just lately been created towards the codebase? Has this problem happened in advance of underneath equivalent conditions? The intention will be to slim down prospects and recognize possible culprits.
Then, test your theories systematically. Seek to recreate the situation in a very controlled ecosystem. In case you suspect a particular functionality or part, isolate it and verify if The difficulty persists. Just like a detective conducting interviews, ask your code thoughts and Permit the results lead you nearer to the truth.
Fork out near notice to smaller information. Bugs frequently cover inside the minimum envisioned spots—like a lacking semicolon, an off-by-1 mistake, or perhaps a race condition. Be extensive and affected person, resisting the urge to patch The difficulty devoid of totally understanding it. Short term fixes may cover the real challenge, only for it to resurface afterwards.
Finally, hold notes on That which you attempted and discovered. Just as detectives log their investigations, documenting your debugging process can help save time for long term challenges and aid Many others understand your reasoning.
By imagining like a detective, developers can sharpen their analytical competencies, method challenges methodically, and become simpler at uncovering concealed concerns in elaborate units.
Create Assessments
Crafting tests is one of the best ways to transform your debugging skills and Total development efficiency. Exams not simply help catch bugs early but in addition function a security Internet that provides you self esteem when earning changes towards your codebase. A well-tested application is easier to debug because it enables you to pinpoint precisely in which and when a difficulty happens.
Begin with unit assessments, which center on particular person capabilities or modules. These smaller, isolated assessments can promptly expose irrespective of whether a selected bit of logic is Performing as predicted. Any time a exam fails, you straight away know where by to glimpse, noticeably cutting down enough time expended debugging. Device exams are Particularly useful for catching regression bugs—concerns that reappear following previously remaining preset.
Following, integrate integration tests and end-to-close assessments into your workflow. These support make certain that a variety of elements of your software get the job done collectively smoothly. They’re significantly handy for catching bugs that take place in complicated units with many elements or services interacting. If something breaks, your assessments can let you know which part of the pipeline unsuccessful and under what ailments.
Creating checks also forces you to definitely think critically regarding your code. To test a attribute appropriately, you will need to know its inputs, predicted outputs, and edge instances. This standard of knowing The natural way potential customers to higher code composition and less bugs.
When debugging a concern, creating a failing test that reproduces the bug might be a powerful initial step. When the test fails persistently, you are able to target correcting the bug and observe your exam pass when The problem is solved. This strategy makes certain that the same bug doesn’t return Later on.
Briefly, writing tests turns debugging from a discouraging guessing game into a structured and predictable approach—serving to you capture far more bugs, a lot quicker and more reliably.
Get Breaks
When debugging a difficult challenge, it’s easy to become immersed in the trouble—watching your display screen for several hours, seeking solution following Remedy. But one of the most underrated debugging resources is just stepping away. Using breaks aids you reset your brain, lessen irritation, and infrequently see The difficulty from the new standpoint.
If you're much too close to the code for as well extended, cognitive tiredness sets in. You could commence overlooking clear problems or misreading code that you just wrote just hrs earlier. Within this state, your brain becomes less productive at difficulty-solving. A short wander, a espresso split, or perhaps switching to a different task for ten–quarter-hour can refresh your emphasis. A lot of developers report discovering the basis of an issue after they've taken the perfect time to disconnect, permitting their subconscious operate inside the background.
Breaks also assistance protect against burnout, Specially in the course of lengthier debugging classes. Sitting down in front of a display, mentally trapped, is not simply unproductive but in addition draining. Stepping away means that you can return with renewed Strength along with a clearer mindset. You may perhaps quickly observe a missing semicolon, a logic flaw, or maybe a misplaced variable that eluded you ahead of.
Should you’re trapped, a very good guideline is to established a timer—debug actively for forty five–60 minutes, then have a 5–ten minute crack. Use that time to maneuver all around, extend, or do anything unrelated to code. It may feel counterintuitive, In particular under restricted deadlines, however it essentially leads to more rapidly and more effective debugging In the long term.
In short, using breaks is not a sign of weak point—it’s a smart approach. It presents your Mind House to breathe, improves your point of view, and allows you avoid the tunnel eyesight That always blocks your progress. Debugging is actually a psychological puzzle, and relaxation is part of fixing it.
Master From Every Bug
Just about every bug you encounter is a lot more than simply a temporary setback—It truly is a possibility to grow like a developer. No matter if it’s a syntax mistake, a logic flaw, or perhaps a deep architectural situation, every one can instruct you some thing useful when you take the time to reflect and examine what went wrong.
Begin by asking by yourself some critical thoughts once the bug is resolved: What brought on it? Why did it go unnoticed? Could it happen to be caught earlier with far better procedures like unit screening, code evaluations, or logging? The solutions usually expose blind places with your workflow or knowledge and assist you Establish much better coding patterns transferring ahead.
Documenting bugs can even be a fantastic routine. Hold a developer journal or sustain a log where you Be aware down bugs you’ve encountered, how you solved them, and Anything you acquired. Over time, you’ll begin to see styles—recurring troubles or frequent blunders—that you could proactively avoid.
In workforce environments, sharing Anything you've figured out from a bug together with your friends is often Specifically potent. Whether or not it’s via a Slack concept, a short create-up, or A fast expertise-sharing session, aiding others steer clear of the very same problem boosts workforce effectiveness and cultivates a more powerful learning lifestyle.
A lot more importantly, viewing bugs as classes shifts your frame of mind from aggravation to curiosity. Instead of dreading bugs, you’ll start out appreciating them as crucial elements of your enhancement journey. All things considered, a few of the most effective developers are usually not the ones who generate best code, but those who repeatedly learn from their problems.
In the end, Every single bug you fix adds a completely new layer in your talent established. So up coming time you squash a bug, take a second to replicate—you’ll come away a smarter, extra capable developer on account of it.
Summary
Enhancing your debugging techniques takes time, follow, and endurance — but the payoff is big. It would make you a far more effective, self-confident, and able developer. Another time you're knee-deep within a mysterious bug, remember: debugging isn’t a chore — it’s an opportunity to become superior at what you do.